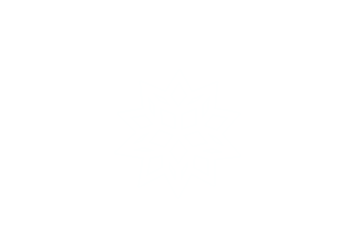
Make Mnemonics for Telephone Numbers
Make telephone numbers easy to remember by finding corresponding words or phrases.

code
how it works
This approach to finding words or phrases that correspond to telephone numbers takes three steps. First make a function that finds single words that correspond to a sequence of digits on a telephone pad. Then make a function that partitions a telephone number into all possible sequences and find words for each sub-sequence. Finally, make a function that forms all combinations of all words for all partitions.
Map digits to the corresponding letters on a telephone pad with a key-value Association. Since 0 and 1 have no letters, associate those digits with themselves:
You can use the Association like a function to map a digit to the corresponding letters:
Map a sequence of digits to the corresponding letters by mapping the Association across the digit list:
To find words that can be formed from those letter choices, construct a regular expression that specifies a sequence of letters, one from each group. Since the dictionary contains both lowercase and uppercase words, make the regular expression contain both lowercase and uppercase letters:
Use DictionaryLookup to find words that match the regular expression:
Package those steps as a function. Since the “words” “0” and “1” are not in the dictionary, add special-case rules for those digits:
Test the function:
The number 4686328 has no telephone word:
But it can be broken into smaller sequences that do have words, for example:
Find all telephone phrases for a list of digits by finding every way of breaking a list of digits into smaller lists and finding words for each smaller list.
Start with a function that finds all partitions of a list into smaller lists. The function is most naturally formulated recursively by thinking about it this way: the partitions of a list are the list itself plus the first n elements of the list combined with all partitions of the remainder of the list, for every n from one less than the length of the list to one:
An n-element list has 2n-1 partitions:
With ListPartition, you can find all telephone word phrases by mapping TelephoneWords across the elements of each partition:
For longer digit lists, however, this approach is prohibitively inefficient. It wastes too much time looking up words later in the list when it already knows that an earlier sublist has no words.
A better approach is to build the TelephoneWords call directly into the recursive function and abort as soon as you know that some sublist has no words. That is what TelephoneWordLists does:
TelephoneWordLists returns lists of lists of words:
To make phrases from the lists of word alternatives, form all combinations of alternative words, for example:
Put hyphens between each word in a sequence using Riffle, and join all the components together into single strings with StringJoin:
Package those steps into a function whose argument is an integer telephone number:
Test the function on a number: