32 | Patterns |
Patterns are a fundamental concept in the Wolfram Language. The pattern _ (read “blank”) stands for anything.
MatchQ tests whether something matches a pattern.
In[1]:=

Out[1]=

{a, b, c} doesn’t match, because it has b in the middle, rather than x:
In[2]:=

Out[2]=

In[3]:=

Out[3]=

In[4]:=

Out[4]=

MatchQ lets you test one thing at a time against a pattern. Cases lets you pick out all the elements (“cases”) in a list that match a particular pattern.
In[5]:=

Out[5]=

Find all elements that match {b, _} (i.e. cases of b followed by something):
In[6]:=

Out[6]=
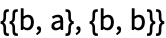
This is what you get if you test whether each element matches {b, _}:
In[7]:=

Out[7]=

In[8]:=

Out[8]=
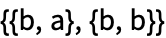
In a pattern, a|b indicates “either a or b”.
Find all cases of either a or b, followed by something:
In[9]:=

Out[9]=

Let’s look at another example, based on creating a list, then picking out elements that match particular patterns.
Create a list by getting the digits of a range of numbers:
In[10]:=

Out[10]=

Find cases that end in 5:
In[11]:=

Out[11]=

Find cases with 1 or 2 in the middle:
In[12]:=

Out[12]=

The notation __ (“double blank”) in a pattern indicates any sequence of things.
Find cases consisting of any sequence, ending with b:
In[13]:=

Out[13]=

Find sequences ending with a or b, or beginning with c:
In[14]:=

Out[14]=

Patterns aren’t just about lists; they can involve anything.
Pick out cases that match the pattern f[_]:
In[15]:=

Out[15]=
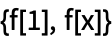
One of the many uses of patterns is to define replacements. /. (“slash dot”) performs a replacement.
Replace b with Red in a list:
In[16]:=

Out[16]=

Replace all occurrences of 2-element lists that begin with 1:
In[17]:=

Out[17]=

You can give a list of replacements to use:
In[18]:=

Out[18]=

The “blank” pattern _ matches absolutely anything. This means, for example, that {_, _} matches any list of two elements. But what if you want to insist that the two elements be the same? You can do that using a pattern like {x_, x_}.
In[19]:=

Out[19]=

In[20]:=

Out[20]=
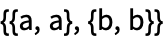
x_ is an example of a named pattern. Named patterns are especially important in replacements, because they give one way to make use of parts of what one’s replacing.
Use the named pattern x_ in a replacement:
In[21]:=

Out[21]=

The form ab is usually called a rule. If x_ appears on the left-hand side of a rule, then whatever the x_ matches can be referred to on the right-hand side as x.
Use x in the right-hand side of the rule to refer to what x_ matches:
In[22]:=

Out[22]=

You can use rules inside Cases as well.
Pick out elements in the list that match f[x_], and give the result of replacing them by x+10:
In[23]:=

Out[23]=
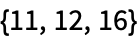
Later on, we’ll see how named patterns are crucial to defining your own functions in the Wolfram Language.
_ | pattern standing for anything (“blank”) | |
__ | pattern standing for any sequence (“double blank”) | |
x_ | pattern named x | |
a|b | pattern matching a or b | |
MatchQ[expr,pattern] | test whether expr matches a pattern | |
Cases[list,pattern] | find cases of a pattern in a list | |
lhsrhs | rule for transforming lhs into rhs | |
expr/.lhsrhs | replace lhs by rhs in expr |
32.1Find lists of length 3 or more beginning with 1 and ending with 9 in IntegerDigits[Range[1000]]. »
32.3In the digit lists for the first 1000 squares, find those that begin with 9 and end with 0 or 1. »
32.6Remove the vowels a, e, i, o and u from the list of characters in “The Wolfram Language”. »
Do the names of pattern variables (x_, etc.) matter?
No. They just have to be consistent inside a given pattern. Different patterns can reuse the same name for different purposes, and the name can also appear outside the pattern.
What else can be used to define patterns?
We’ll discuss several more things in Section 41.
p |q is a pattern construct, that matches either p or q. p ||q is a logic construct, that tests whether p or q is True.
How does one say a|b?
Either “a or b” or “a vertical bar b”.
How is /. interpreted?
It’s the function ReplaceAll. Replace tries to replace a whole expression. ReplaceList gives a list of results from all possible ways to match a particular pattern.
If /. has several replacements, which one will it use?
It uses the first one that applies. If replacements apply to multiple levels of an expression, /. will use it on the outermost level.
- Patterns for strings are discussed in Section 42.
- Pattern matching in the Wolfram Language takes account of facts such as the equivalence of x+y and y+x, or x +(y +z) and (x +y)+z. See Section 41.
- In writing lhsrhs, the lhs stands for “left-hand side” and the rhs for “right-hand side”.
- Patterns are scoping constructs, in the sense that they localize names like the x in x_ to just the scope of the pattern.
- In the rare case that /. is followed by a digit (like 0), you need to leave a space before the digit to avoid confusion with division.