31 | Parts of Lists |
Part lets you pick out an element of a list.
Pick out element 2 from a list:
In[1]:=

Out[1]=

[[...]] is an alternative notation.
Use [[2]] to pick out element 2 from a list:
In[2]:=

Out[2]=

Negative part numbers count from the end of a list:
In[3]:=

Out[3]=

You can ask for a list of parts by giving a list of part numbers.
Pick out parts 2, 4 and 5:
In[4]:=

Out[4]=

;; lets you ask for a span or sequence of parts.
Pick out parts 2 through 5:
In[5]:=

Out[5]=
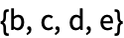
Take the first 4 elements from a list:
In[6]:=

Out[6]=
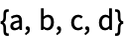
Take the last 2 elements from a list:
In[7]:=

Out[7]=

In[8]:=

Out[8]=
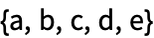
Let’s now talk about lists of lists, or arrays. Each sublist acts as a row in the array.
In[9]:=

Out[9]=
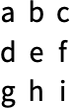
Pick out the second sublist, corresponding to the second row, in the array:
In[10]:=

Out[10]=

This picks out element 1 on row 2:
In[11]:=

Out[11]=

It’s also often useful to pick out columns in an array.
Pick out the first column, by getting element 1 on all rows:
In[12]:=

Out[12]=

The function Position finds the list of positions at which something appears.
Here there’s only one d, and it appears at position 2, 1:
In[13]:=

Out[13]=

This gives a list of all positions at which x appears:
In[14]:=

Out[14]=

The positions at which “a” occurs in a list of characters:
In[15]:=

Out[15]=

In[16]:=

Out[16]=

The function ReplacePart lets you replace parts of a list:
Replace part 3 with x:
In[17]:=

Out[17]=

Replace two parts:
In[18]:=

Out[18]=

Replace 5 randomly chosen parts with “--”:
In[19]:=

Out[19]=

Sometimes one wants particular parts of a list to just disappear. One can do this by replacing them with Nothing.
Nothing just disappears:
In[20]:=

Out[20]=
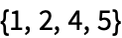
Replace parts 1 and 3 with Nothing:
In[21]:=

Out[21]=
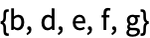
Take 50 random words, dropping ones longer than 5 characters, and reversing others:
In[22]:=

Out[22]=

Take takes a specified number of elements in a list based on their position. TakeLargest and TakeSmallest take elements based on their size.
Take the 5 largest elements from a list:
In[23]:=

Out[23]=

From the first 100 Roman numerals take the 5 that have the largest string length:
In[24]:=

Out[24]=

Part[list,n] | part n of a list | |
list[[n]] | short notation for part n of a list | |
list[[{n1,n2,...}]] | list of parts n1, n2, ... | |
list[[n1;;n2]] | span (sequence) of parts n1 through n2 | |
list[[m,n]] | element from row m, column n of an array | |
list[[All,n]] | all elements in column n | |
Take[list,n] | take the first n elements of a list | |
TakeLargest[list,n] | take the largest n elements of a list | |
TakeSmallest[list,n] | take the smallest n elements of a list | |
TakeLargestBy[list,f,n] | take elements largest by applying f | |
TakeSmallestBy[list,f,n] | take elements smallest by applying f | |
Position[list,x] | all positions of x in list | |
ReplacePart[list,nx] | replace part n of list with x | |
Nothing | a list element that is automatically removed |
31.1Find the last 5 digits in 2^1000. »
31.2Pick out letters 10 through 20 in the alphabet. »
31.3Make a list of the letters at even-numbered positions in the alphabet. »
31.4Make a line plot of the second to last digit in the first 100 powers of 12. »
31.5Join lists of the first 20 squares and cubes, and get the 10 smallest elements of the combined list. »
31.6Find the positions of the word “software” in the Wikipedia entry for “computers”. »
31.8Make a list of the first 100 cubes, with every one whose position is a square replaced by Red. »
31.9Make a list of the first 100 primes, dropping ones whose first digit is less than 5. »
31.10Make a grid starting with Range[10], then at each of 9 steps randomly removing another element. »
31.12Find the 5 longest integer names for integers up to 100. »
31.13Find the 5 English names for integers up to 100 that have the largest number of “e”s in them. »
How does one read list[[n]] out loud?
Usually “list part n” or “list sub n”. The second form (with “sub” short for “subscript”) comes from thinking about math and extracting components of vectors.
What happens if one asks for a part of a list that doesn’t exist?
One gets a message, and the original computation is returned undone.
Can I just get the first position at which something appears in a list?
Yes. Use FirstPosition.
How can I insert a sequence of elements into a list?
A convenient way is to replace one element with Splice[{x, y, ...}], which automatically “splices itself” in, so that for example {a, b, Splice[{x, y}], c} gives {a, b, x, y, c}.